When writing code in C++ in order to execute in, it needs to be converted multiple times by different processes.

Once the .cpp files and .h files are ready, the preprocessor is going to make substitutions in the code to make little edits and adjustments. The output of the preprocessor phase is still a C++ code.
The compiler takes over next: it uses the preprocessor-edited code and translates the C++ code into machine code. One of the compiler’s jobs is to also optimize the code that was written.
The last phase is in the linker’s hands, as it links together all the compiled files and turns them into an executable that can be run on that machine.
Functions in C++
When writing functions in C++ we have to follow the structure reported below
int f(int i); // Function declaration
int main(void) {
int i = 0;
i = f(i); // Function call
return 0;
}
int f(int i) {
return i+2; // function definition
}
In case we want to distribute such code into multiple files, we could insert the function declaration into a header file that we can call my_func.h
, while the function definition can be placed inside a library source file that we can call my_func.cpp
.
The steps required to translate the code (which could be split into different files) in therefore the following:
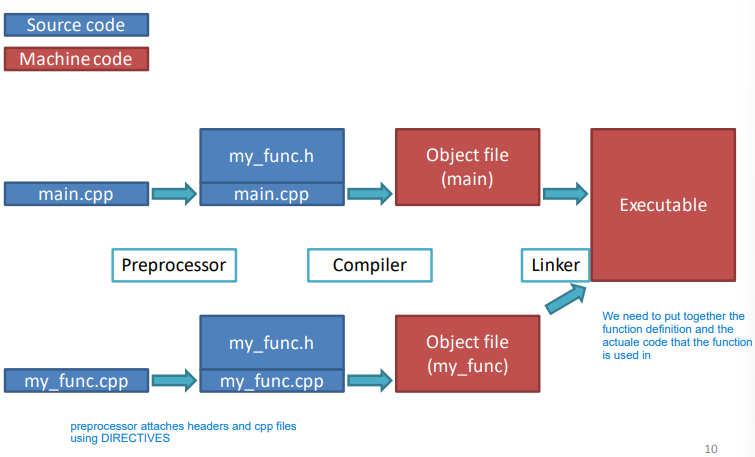
External libraries
If we want to use external libraries in our project, the files that contain the source code for those libraries have to be merged together with our own following the same steps specified above. For example, in the following code
#include <iostream>
int main(void) {
std::cout << "Hello world\n";
return 0;
}
The code is merged together in the following way
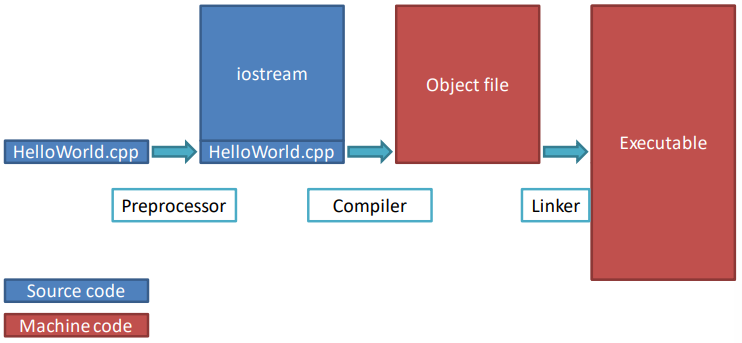
Static vs. dynamic libraries
When we use static libraries the source code of such library is appended to our own source code in every file, instead, by using dynamic libraries, the same file can be dynamically attached to all object files at the same time.

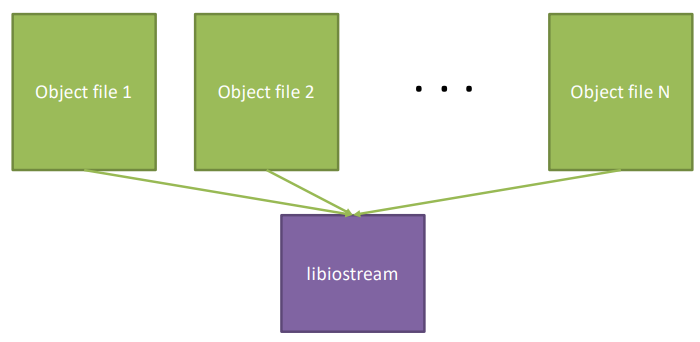
Therefore, the following properties of dynamic libraries are true:
- They save disk space
- They can be recompiled without touching the executables
- They are called SO (shared object) in Linux, while DLL on Windows
For static libraries instead we have:
- They generate execs that can’t be broken at a later stage
- They are self contained
The standard library
The C++ standard library provides supporto for strings, I/O streams, memory management, threads, math, and a lot of other things. One of the main concepts that is used behind the development of the standard library is the template. Templates are a set of conventions that support generic containers like vectors, lists, sets and queues.
Templates are converted into the appropriate code by the compiler at compile-time, so for example, the following function
template <typename T>
T f(T t) {
return t;
}
So we can use the following function calls with no error.
int i;
float f;
f<int> (i);
f<float> (f);
Pointers
Setting values using pointers:
// Declare a pointer and assign the address of myVariable to it
int* myPointer = &myVariable;
// Set the value at the memory location pointed to by myPointer
*myPointer = 99;
Reading values using pointers:
int i;
fl// Declare a pointer and assign the address of myVariable to it
int* myPointer = &myVariable;
// Read the value at the memory location pointed to by myPointer
int readValue = *myPointer;
References
References create aliases to other variables
int i = 10;
// Declare a reference 'r' to the integer variable 'i'.
// The reference 'r' is another name for the same memory location as 'i'.
int &r = i;
// Assign the value 15 to the reference 'r'.
// Since 'r' is a reference to 'i', this also changes the value of 'i'.
r = 15;
Comparing the two things
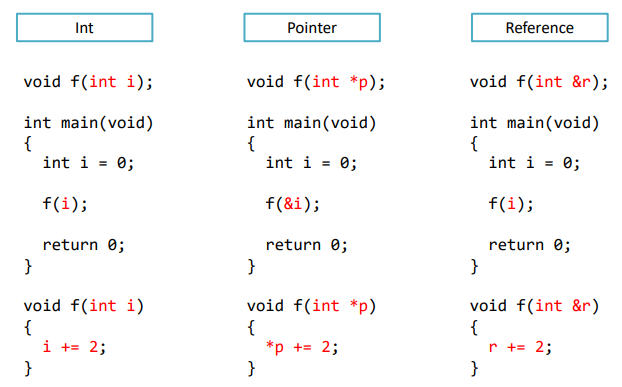
Casts
We can convert one variable type into another, but this could cause loss of information.
We can also uso the void*
pointer, which is generic and might be requested by OpenCV.
Callbacks
A callback is any executable code that is passed as an argument to other code that is expected to call back the argument at a given time. OpenCV can handle callbacks, for example with the mouse or some GUI elements.